먼저 다음과 같이 CuratorFramework 인스턴스를 빌드해야 합니다. 여기서 connectString은 앙상블에 있는 서버의 IP 및 포트 조합이 쉼표로 구분된 목록을 의미합니다.
CuratorFrameworkFactory.Builder builder = CuratorFrameworkFactory.builder()
.connectString(connectString)
.retryPolicy(new ExponentialBackoffRetry(retryInitialWaitMs, maxRetryCount))
.connectionTimeoutMs(connectionTimeoutMs)
.sessionTimeoutMs(sessionTimeoutMs);
/*
* If authorization information is available, those will be added to the client. NOTE: These auth info are
* for access control, therefore no authentication will happen when the client is being started. These
* info will only be required whenever a client is accessing an already create ZNode. For another client of
* another node to make use of a ZNode created by this node, it should also provide the same auth info.
*/
if (zkUsername != null && zkPassword != null) {
String authenticationString = zkUsername + ":" + zkPassword;
builder.authorization("digest", authenticationString.getBytes())
.aclProvider(new ACLProvider() {
@Override
public List<ACL> getDefaultAcl() {
return ZooDefs.Ids.CREATOR_ALL_ACL;
}
@Override
public List<ACL> getAclForPath(String path) {
return ZooDefs.Ids.CREATOR_ALL_ACL;
}
});
}
객체 빌드 및 시작해야 합니다.
CuratorFramework client = builder.build();
client.start();
Creating a ZNode
client.create().withMode(CreateMode.PERSISTENT).forPath("/your/ZNode/path");
여기에서 CreateMode는 생성하려는 노드 유형을 지정합니다. 사용 가능한 유형은 PERSISTENT, EPHEMERAL, EPHEMERAL_SEQUENTIAL, PERSISTENT_SEQUENTIAL, CONTAINER입니다.
(https://zookeeper.apache.org/doc/r3.5.1-alpha/api/org/apache/zookeeper/CreateMode.html)
/your/ZNode까지의 경로가 이미 존재하는지 확실하지 않은 경우 경로를 생성할 수도 있습니다.
client.create().creatingParentsIfNeeded().withMode(CreateMode.PERSISTENT).forPath("/your/ZNode/path");
Set Data
ZNode를 생성할 때 또는 나중에 데이터를 설정할 수 있습니다. 생성 시 데이터를 설정하는 경우 해당 데이터를 forPath() 메서드의 두 번째 매개 변수로 바이트 배열로 전달합니다.
client.create().withMode(CreateMode.PERSISTENT).forPath("/your/ZNode/path","your data as String".getBytes());
나중에 하게 된다면 (데이터는 바이트 배열로 주어야 함)
client.setData().forPath("/your/ZNode/path",data);
Finally
Apache Curator는 Apache Zookeeper를 백그라운드에서 사용하고 Zookeeper의 엣지 케이스와 복잡성을 숨기는 Java 클라이언트(Curator Recipes보다 더 많은)입니다. Zookeeper에서는 ZNode 개념을 사용하여 데이터를 저장합니다. 모든 ZNodePath는 /(루트)로 시작해야 하며 원하는 대로 ZNodePaths와 같은 디렉터리를 계속 지정할 수 있습니다. 예: /someName/another/test/sample.
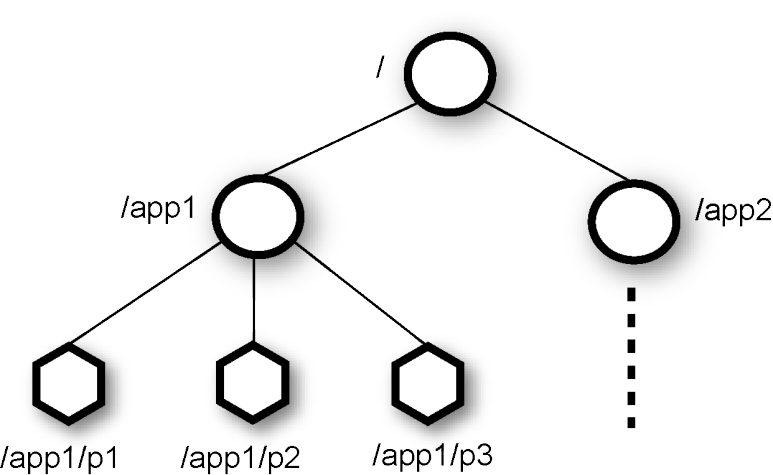
ZNODE에 대한 설명은 다음 블로그를 참고 바랍니다.
https://blog.naver.com/mlljs1998/223413635483
특정 경로에서 데이터를 검색하려면, 다음과 같이 수행할 수 있습니다.
client.getData().forPath("/path/to/ZNode");
One more thing
Apache Curator의 ACL은 액세스 제어를 위한 것입니다. 따라서 ZooKeeper는 올바른 비밀번호가 없는 클라이언트는 ZooKeeper에 연결할 수 없거나 ZNode를 생성할 수 없는 것과 같은 인증 메커니즘을 제공하지 않습니다. 이것이 할 수 있는 일은 승인되지 않은 클라이언트가 특정 Znode/ZNodes에 액세스하는 것을 방지하는 것입니다. 그렇게 하려면 아래에 설명된 대로 CuratorFramework 인스턴스를 설정해야 합니다. 이는 지정된 ACL을 사용하여 생성된 ZNode가 동일한 클라이언트 또는 동일한 인증 정보를 제공하는 클라이언트에 의해 다시 액세스될 수 있음을 보장한다는 점을 기억하십시오.
ACLProvider를 다음과 같이 설정하면,
new ACLProvider() {
@Override
public List<ACL> getDefaultAcl () {
return ZooDefs.Ids.CREATOR_ALL_ACL;
}
@Override
public List<ACL> getAclForPath (String path){
return ZooDefs.Ids.CREATOR_ALL_ACL;
}
}
나중에 작성자와 동일한 자격 증명을 가진 클라이언트에게만 해당 ZNode에 대한 액세스 권한이 부여됩니다. 인증 세부 사항은 다음과 같이 설정됩니다. (클라이언트 구축 예시 참조) ACLProvider로 설정하면 액세스 제어를 수행하지 않는 OPEN_ACL_UNSAFE와 같은 다른 ACL 모드도 사용할 수 있습니다.
나중에 특정 ZNode에 대한 액세스를 제어하는데 사용됩니다.
즉, 다른 사람이 ZNode를 방해하지 못하도록 하려면 ACLProvider가 CREATOR_ALL_ACL을 반환하도록 설정하고 위에 표시된 대로 다이제스트에 대한 인증을 설정할 수 있습니다. 동일한 인증 문자열("사용자 이름:비밀번호")을 사용하는 CuratorFramework 인스턴스만 해당 ZNode에 액세스할 수 있습니다. 하지만 다른 사람이 자신의 경로를 방해하지 않는 경로에 ZNode를 만드는 것을 막지는 못합니다.
'Bigdata > Zookeeper' 카테고리의 다른 글
Zookeeper Mac 설치 및 실행 (0) | 2024.05.28 |
---|---|
Zookeeper 개념 및 아키텍처 (0) | 2024.05.21 |